Introduction
In the past, I have struggled to create custom findings in AWS GuardDuty or GCP Security Command Center (SCC). GCP SCC has an amazing feature to create custom findings via the API. This feature is quite hidden in the documentation, but in this article, we will explore the different possibilities.
GCP SCC is a powerful tool that allows you to monitor and analyze your cloud infrastructure's security posture. 🛡️
Benefits of Custom Security Findings
Creating custom security findings in SCC offers several advantages. Firstly, it enables you to define security rules and policies that align with your organization's unique requirements and compliance standards. This flexibility ensures that you can address specific security concerns effectively. 🔒
Moreover, custom findings allow you to incorporate additional data sources beyond the default set provided by GCP. By leveraging logs, events, and metrics from your applications and infrastructure, you can gain deeper insights into the security posture of your environment. 📊
This article will explore how to effectively create custom GCP SCC findings in Node.js. 🔍
Prerequisites
- Node.js
- Security Command Center API
- Install
npm install @google-cloud/security-center
- IAM permission
securitycenter.findingsEditor
Create a new SCC source
First, we create a new source for our custom findings. We need to note down the name
as we need to later for creating the finding.
const {SecurityCenterClient} = require('@google-cloud/security-center');
const client = new SecurityCenterClient();
const organizationId = "1234567890";
async function createSource() {
const [source] = await client.createSource({
source: {
displayName: 'Custom Event Source',
description: 'A new custom source that collects events',
},
parent: client.organizationPath(organizationId),
});
console.log('New Source: %j', source);
}
createSource();
New Source:
{
"name":"organizations/1234567890/sources/1234567890123456789",
"displayName":"Custom Event Source",
"description":"A new custom source that collects events",
"canonicalName":""
}
List all SCC source
Alternatively, you can also list all SCC sources with the following code:
const {SecurityCenterClient} = require('@google-cloud/security-center');
const client = new SecurityCenterClient();
const organizationId = "1234567890";
const orgName = client.organizationPath(organizationId);
async function listSources() {
const [response] = await client.listSources({parent: orgName});
console.log('Sources:');
Array.from(response).forEach(source =>
console.log('%s: %s', source.displayName, source.name)
);
}
listSources();
Custom Event Source: organizations/1234567890/sources/1234567890123456789
Event Threat Detection Custom Modules: organizations/1234567890/sources/12345678901234567891
Container Threat Detection: organizations/1234567890/sources/12345678901234567892
[...]
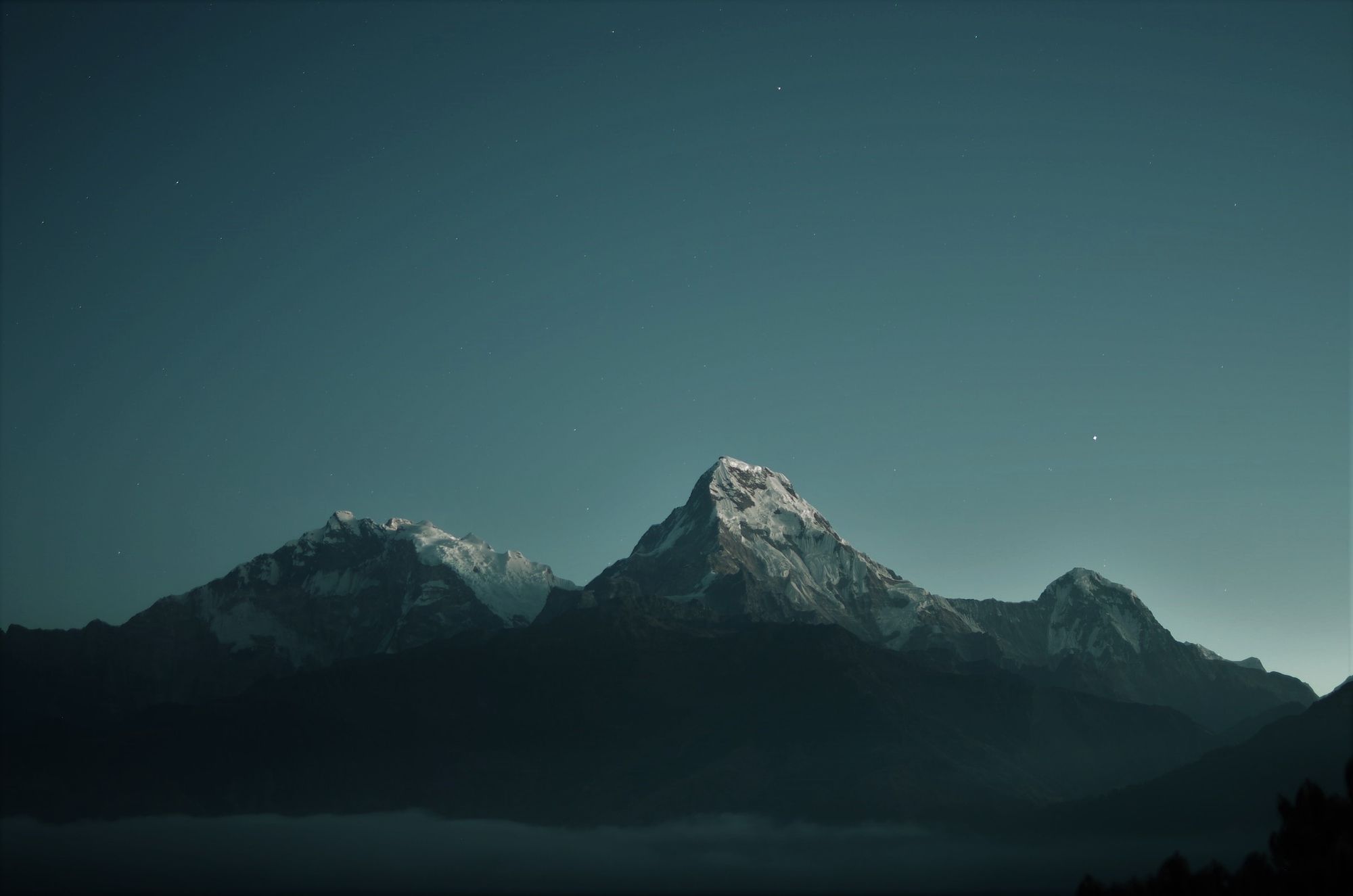
Join our community of cloud security professionals. 🔐
Subscribe to our newsletterCreate findings in SCC
With the SCC API, you can start defining custom findings within your Node.js application. This includes specifying the finding details, such as state, name, severity, category, and affected resource, ... Make sure to assign each finding a unique ID as the findingId
.
const {SecurityCenterClient} = require('@google-cloud/security-center');
const { v4: uuidv4 } = require('uuid');
const i = uuidv4();
const client = new SecurityCenterClient();
const sourceName = "organizations/1234567890/sources/1234567890123456789";
const eventTime = new Date();
async function createFinding() {
const [newFinding] = await client.createFinding({
parent: sourceName,
findingId: uuidv4().replaceAll('-', ''),
finding: {
state: 'ACTIVE',
resourceName: '//cloudresourcemanager.googleapis.com/organizations/1234567890',
severity: "HIGH",
category: 'CREDENTIAL_EXFILTRATION',
eventTime: {
seconds: Math.floor(eventTime.getTime() / 1000),
nanos: (eventTime.getTime() % 1000) * 1e6,
},
externalUri: "https://alexanderhose.com",
},
});
console.log('New finding created: %j', newFinding);
}
createFinding();
Afterward, we will see the result in the UI of SCC 🎉
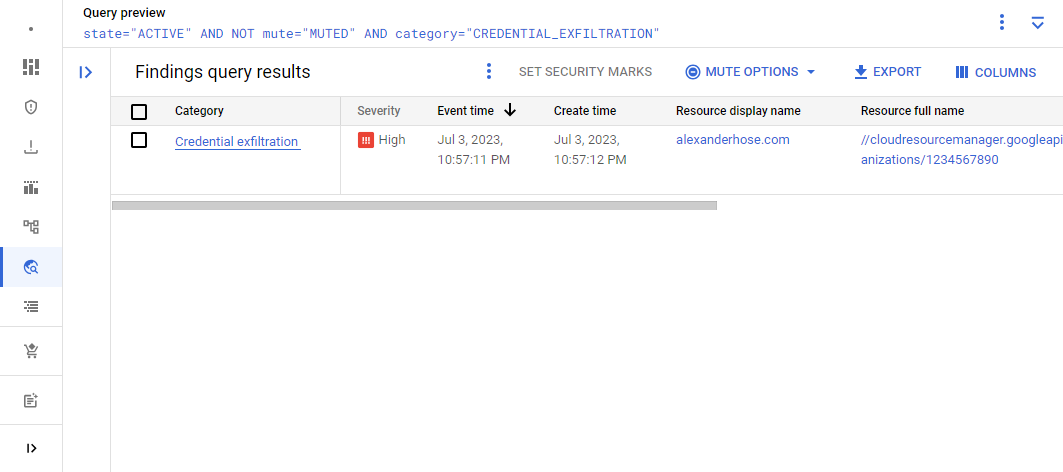
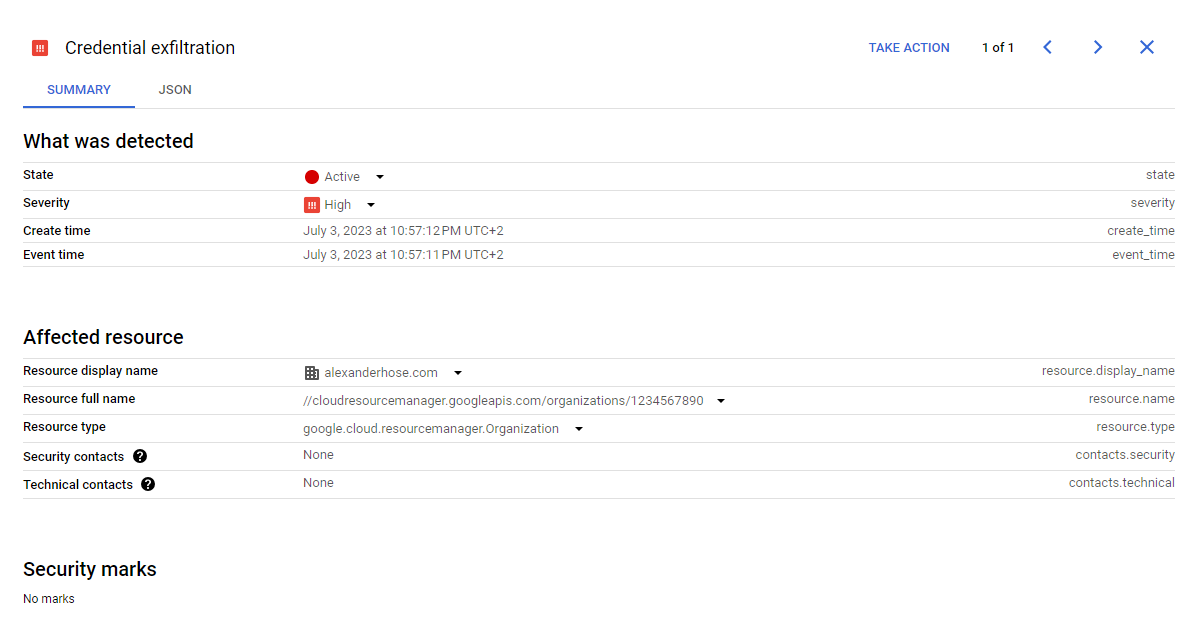
We could also add more information to the finding based on our use case. The quick filters will automatically adjust with the information from the different findings.
Conclusion 💭
Creating custom GCP Security Command Center findings empowers organizations to tailor their security monitoring and response capabilities to their specific needs. By leveraging the flexibility and customization options provided by the Security Command Center, you can enhance the security posture of your GCP environment.
Member discussion